目次
目次
動く床
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MoveFloor : MonoBehaviour
{
private Vector3 initialPosition;
[SerializeField]
private int movex = 0;
[SerializeField]
private int movey = 0;
[SerializeField]
private int movez = 0;
[SerializeField]
private int movespeed = 0;
// Start is called before the first frame update
void Start()
{
initialPosition = transform.position;
}
// Update is called once per frame
void Update()
{
transform.position = new Vector3(Mathf.Sin(Time.time * movespeed) * movex + initialPosition.x, Mathf.Sin(Time.time * movespeed) * movey + initialPosition.y, Mathf.Sin(Time.time * movespeed) * movez + initialPosition.z);
}
}
設定
- ①動かしたいオブジェクトにスクリプト、コライダーをつける
- ②Move x,y,zに数字を入力して、移動する方向や距離を決める
※+-で方向が変わります
- ③MoveSpeedに数字を入力して、移動する速さを決める
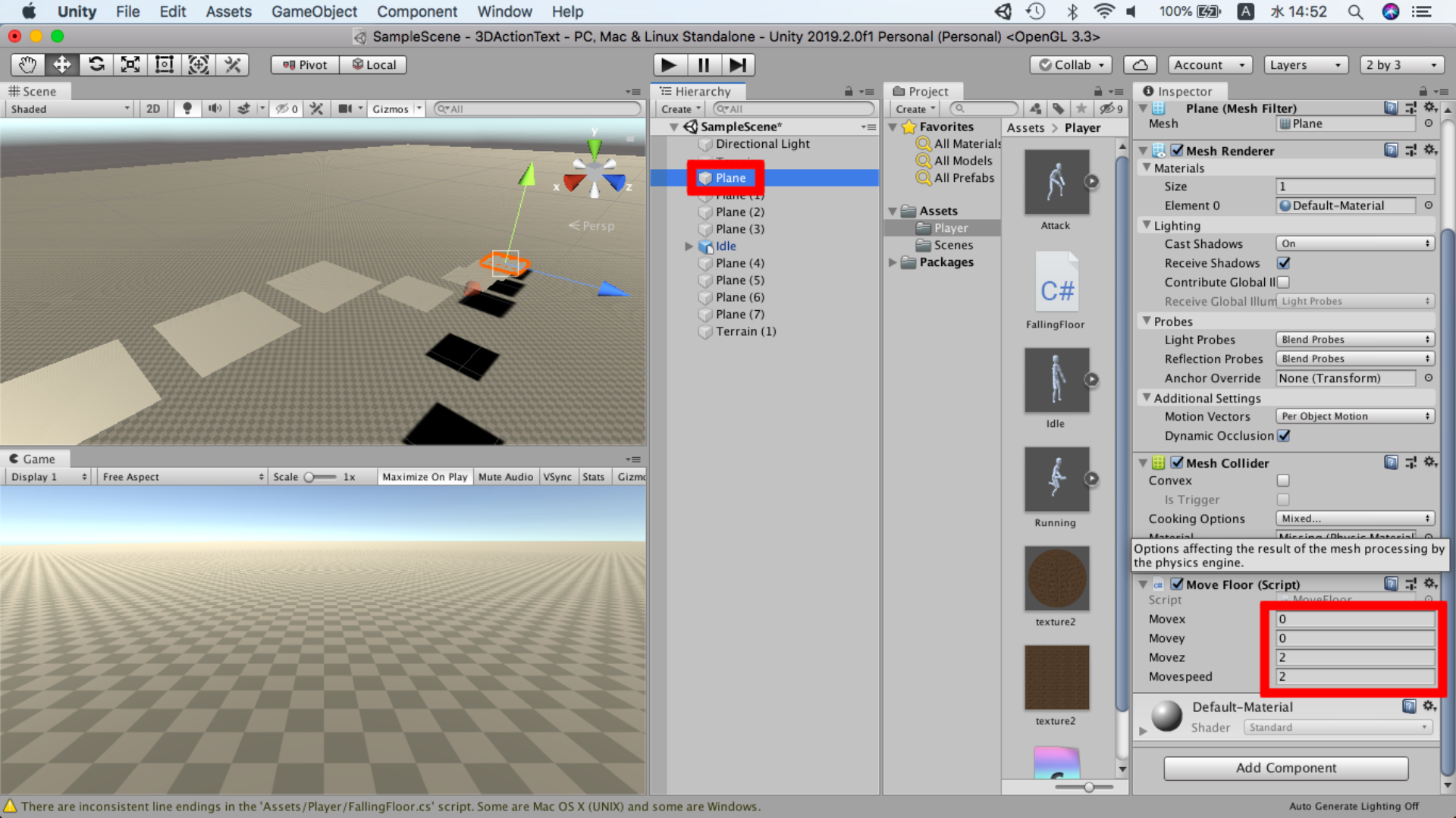
触れると落ちる床
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FallingFloor : MonoBehaviour
{
private Vector3 initialPosition;
private bool Falling;
private int FallingSpeed = 10;
// Start is called before the first frame update
void Start()
{
initialPosition = transform.position;
}
// Update is called once per frame
void Update()
{
if(Falling == true)
{
transform.position -= new Vector3(0, FallingSpeed * Time.deltaTime, 0);
}
if(transform.position.y <= -10)
{
transform.position = initialPosition;
Falling = false;
}
}
private void OnCollisionEnter(Collision collision)
{
if(collision.gameObject.tag == "Player")
{
Falling = true;
}
}
}
設定
- ①動かしたいオブジェクトにスクリプト、コライダーをつける
- ②プレイヤーにRigidbodyとコライダーをつける
※RigidbodyのFreezeRotationにはチェックを入れておきましょう
- ③プレイヤーにPlayerというタグをつける
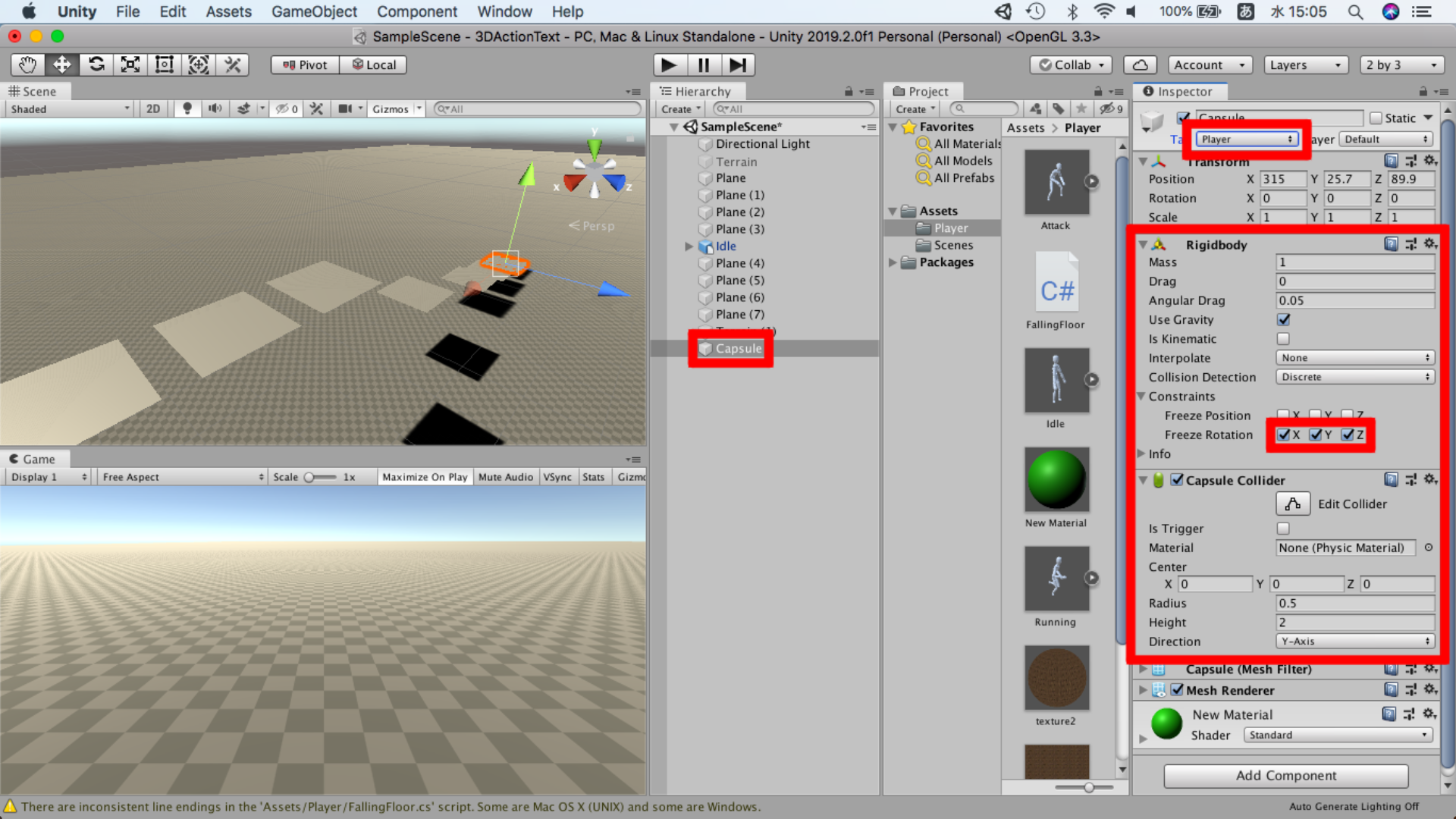
床が動いた時においていかれないようにする
using UnityEngine;
using System.Collections;
public class notfall : MonoBehaviour
{
void OnCollisionEnter(Collision col) {
if (col.gameObject.tag == "MoveFloor") {
transform.SetParent(col.transform);
}
}
void OnCollisionExit(Collision col) {
if (col.gameObject.tag == "MoveFloor") {
transform.SetParent(null);
}
}
}
設定
- ①動く床、落ちる床にMoveFloorというタグをつける
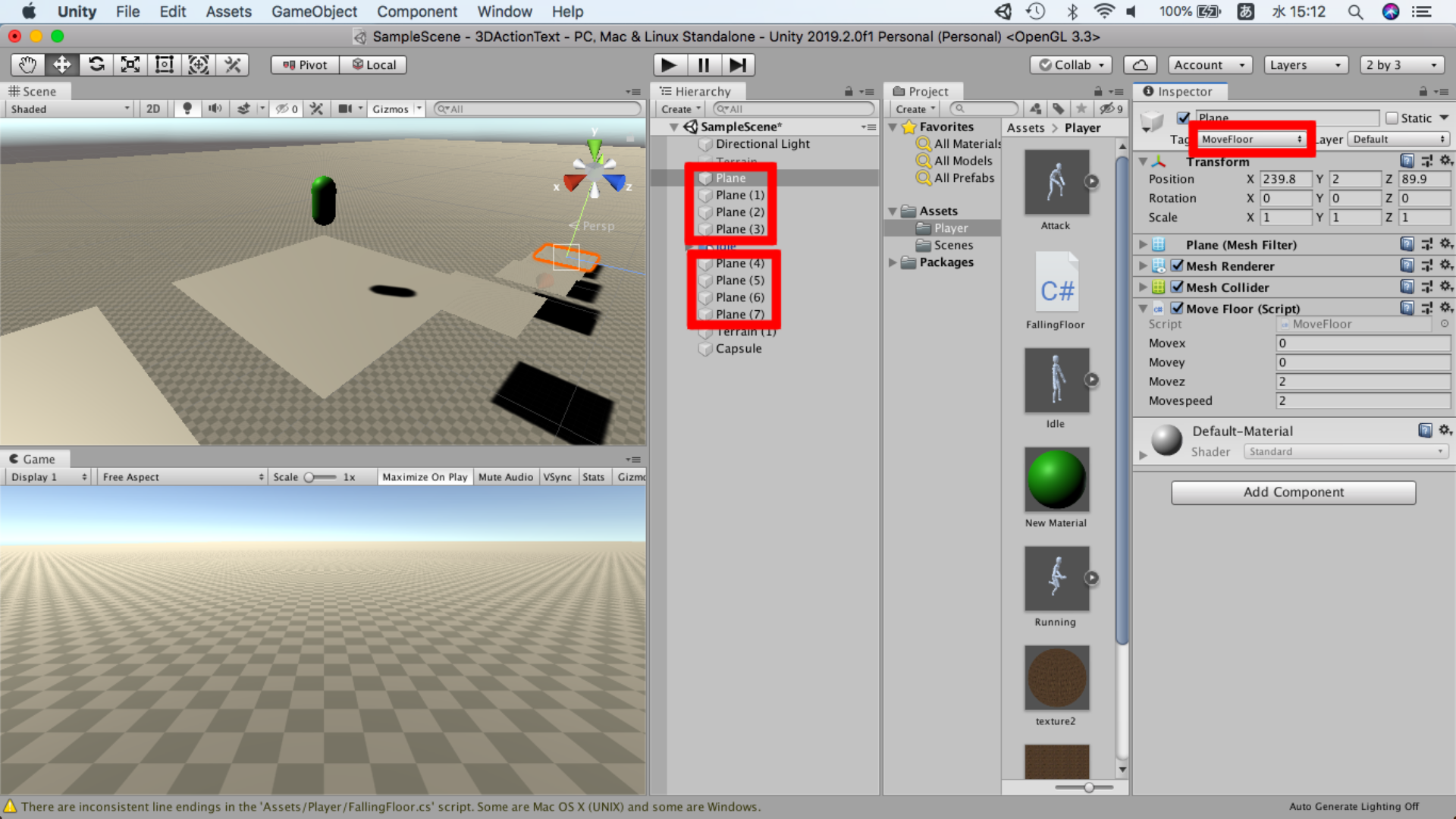
コメント